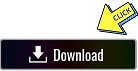
The crucial part of this implementation is that after consuming a Stream instance, we end up with a modified queue – the poll() method removes the polled element from the queue. Until Java 9 gets released, we can’t terminate the generated Stream dynamically so we need to rely on limiting the Stream size to the queue size – which is not perfect because this can change during an actual processing. The problem with this implementation is that it’s not concurrent-modification-friendly. Instead of locks, this queue uses CAS (Compare-And-Swap).

Consequently, it provides a wait-free algorithm where add and poll are guaranteed to be thread-safe and return immediately. The Java Queue provides support for all of the methods of the Collection interface including deletion, insertion, etc. containsExactly("1", "22", "333", "4444", "55555") The ConcurrentLinkedQueue is the only non-blocking queue of this guide.
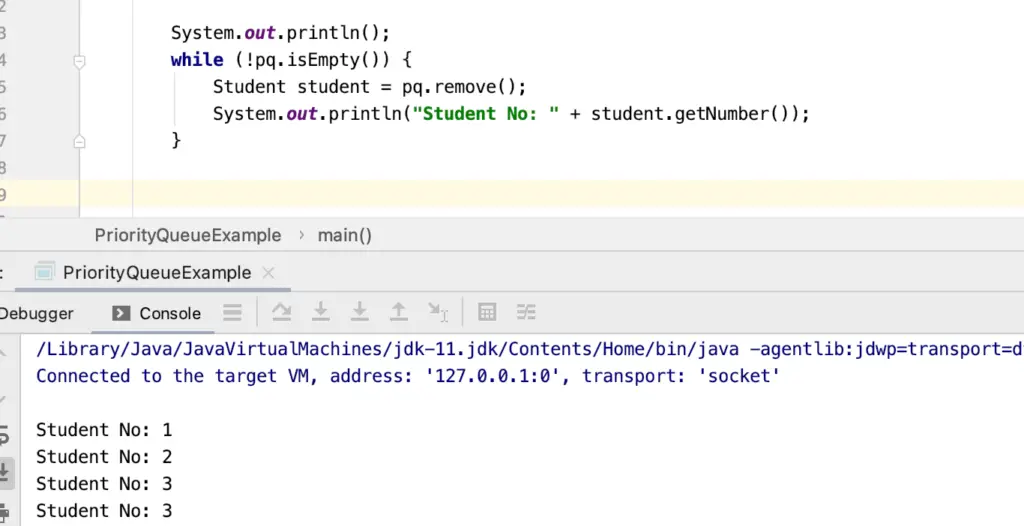
So – let’s generate a Stream from consecutive elements, returned by the poll() method, using Stream.generate() method: List result = Stream.generate(queue::poll) construct an empty queue enqueue: add an element somewhere in the queue (at the back of a normal queue, according to prirority in a priority queue) dequeue. We can use the poll() method to fetch elements from the queue according to the priority order. We can see that the insertion order gets preserved regardless of the fact that our queue was expected to be providing Strings according to their length. The same behaviour can be noticed when trying to use Java Stream API for processing PriorityQueue’s elements using the instance obtained by the stream() method – the Stream instance depends on the Spliterator instance which doesn’t guarantee the desired traversal order: PriorityQueue queue = new PriorityQueue(comparing(String::length)) The iterator does not return the elements in any particular order. Priority Queue has some priority assigned to each element, The element with Highest priority appears at the Top Of Queue.
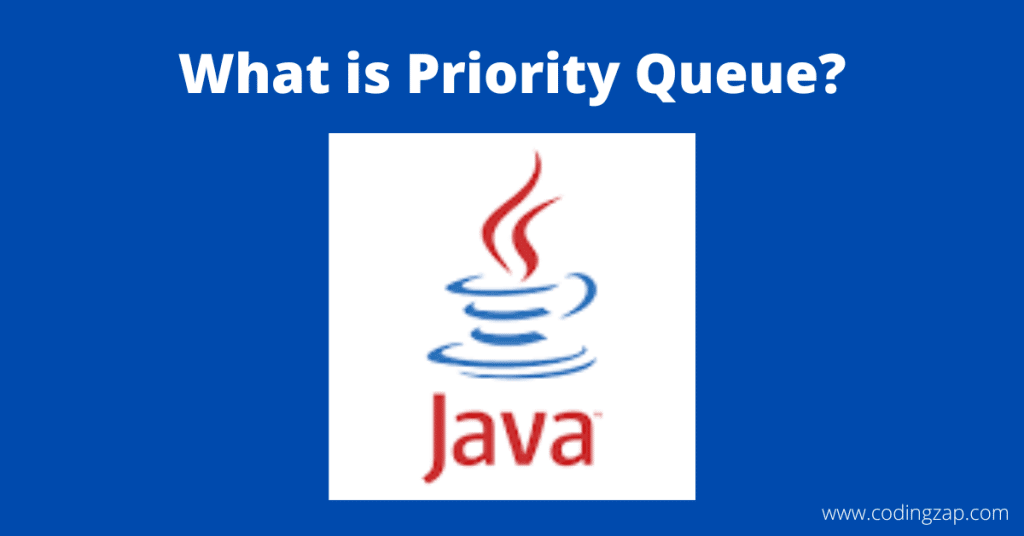
Returns an iterator over the elements in this queue. If we have a look at erator() documentation, we’ll see that, unintuitively, iterator() is not traversing the queue according to its priority order: I was recently reminded that Java has priority queues as part of its collections framework. The tricky thing about working with PriorityQueues is that, ironically, they don’t always behave according to the PriorityQueue semantics.
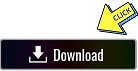